User Control Arrays
Prerequisites
Lecture – Form Objects – User Controls
Summary
Demonstrates how to create a complex user control and then use it as a control array inside of another user control. Very useful coding pattern for handling forms with patterns.
Video
Video for this lecture has not been produced yet.
Reference Materials
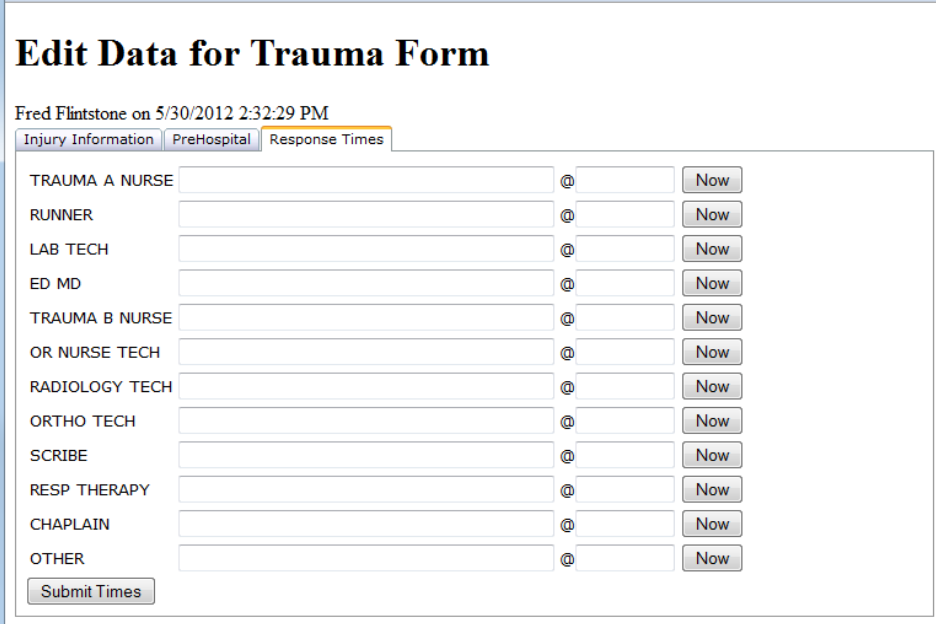
The user control that represents a single line in the form is ucStaffResponseTime. The Control Array is part of the container control which is ucTraumaResponseTimes. This user control is then placed on pages as needed.
ucStaffResponseTime.ascs |
<%@ Control Language="C#" AutoEventWireup="true" CodeBehind="ucStaffResponseTime.ascx.cs" Inherits="TraumaFlow.UserControls.ucStaffResponseTime" %>
<%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit" TagPrefix="cc1" %>
<tr>
<td class="TableLeft"><asp:Label ID="lblJob" runat="server" Text=""></asp:Label></td>
<td class="TableRight"><asp:TextBox ID="tbStaff" runat="server" Width="300"></asp:TextBox>
<cc1:AutoCompleteExtender ID="aceStaff" runat="server"
TargetControlID="tbStaff"
ServiceMethod="names"
MinimumPrefixLength="1" >
</cc1:AutoCompleteExtender>
</td>
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<td>@<asp:TextBox ID="tbTime" runat="server" Width="75"></asp:TextBox>
<asp:Button ID="btnNow" runat="server" Text="Now" onclick="btnNow_Click"></asp:Button></td>
</ContentTemplate>
</asp:UpdatePanel>
</tr>
ucStaffResponseTime.ascx.cs |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using TraumaFlow.WebServices;
using TraumaFlow.Code;
namespace TraumaFlow.UserControls
{
public partial class ucStaffResponseTime : System.Web.UI.UserControl
{
public int TraumaFormID()
{
if (Session["TraumaFormID"] == null)
return 0;
else
return Convert.ToInt32(Session["TraumaFormID"]);
}
public string StaffName
{
get { return tbStaff.Text; }
set { tbStaff.Text = value; }
}
public string Label
{
get { return lblJob.Text; }
set { lblJob.Text = value; }
}
public string Time
{
get { return tbTime.Text; }
set { tbTime.Text = value; }
}
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnNow_Click(object sender, EventArgs e)
{
tbTime.Text = string.Format("{0:HH:mm}", DateTime.Now);
if (StaffName != String.Empty)
{
// Data is Saved as Role;Name;Time
string s = this.ID;
s += ";" + StaffName + ";" + Time;
TraumaFlowHelper.updateMedicalData(TraumaFormID(), "TraumaCallStaffAndResponseTime", s);
}
}
[System.Web.Services.WebMethodAttribute(), System.Web.Script.Services.ScriptMethod()]
public static string[] names(string pattern)
{
FormServices fs = new FormServices();
return fs.StaffNames(pattern);
}
}
}
ucTraumaResponseTime.ascx |
<%@ Control Language="C#" AutoEventWireup="true" CodeBehind="ucTraumaResponseTimes.ascx.cs" Inherits="TraumaFlow.UserControls.ucTraumaResponseTimes" %>
<div class="TraumaResponseControls">
<table>
<asp:PlaceHolder ID="ph1" runat="server"></asp:PlaceHolder>
</table>
</div>
<asp:Button ID="btnSubmit" runat="server" Text="Submit Times"
onclick="btnSubmit_Click" />
ucTraumaResponseTime.ascx.cs |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using TraumaFlow.Code;
namespace TraumaFlow.UserControls
{
public partial class ucTraumaResponseTimes : System.Web.UI.UserControl
{
private ucStaffResponseTime[] staffControls;
public int TraumaFormID()
{
if (Session["TraumaFormID"] == null)
return 0;
else
return Convert.ToInt32(Session["TraumaFormID"]);
}
protected void Page_Load(object sender, EventArgs e)
{
staffControls = new ucStaffResponseTime[30];
int i = 1;
foreach (string s in TraumaFlowHelper.traumaTeamMembers())
{
string s2 = s.Replace('_', ' ');
staffControls[i] = (ucStaffResponseTime)LoadControl("~/UserControls/ucStaffResponseTime.ascx");
staffControls[i].Label = s2;
staffControls[i].ID = s;
ph1.Controls.Add(staffControls[i]);
i++;
}
}
protected void btnSubmit_Click(object sender, EventArgs e)
{
foreach (ucStaffResponseTime u in ph1.Controls)
{
if (u.StaffName != String.Empty)
{
// Data is Saved as Role;Name;Time
string s = u.ID;
s += ";" + u.StaffName + ";" + u.Time;
TraumaFlowHelper.updateMedicalData(TraumaFormID(), "TraumaCallStaffAndResponseTime", s);
}
}
}
}
}
Additional Information
This video is part of Case Study – Trauma Flow Database