The MyDailyMath Equation Editor
Prerequisites
Lecture – Advanced – User Control Arrays
Summary
Demonstration of the code of the MydailyMath equation editor – showing the use of User Controls, Html Text Box (Ajax Control), and Math ML embedded in a literal control to show mathematics equations.
Video
Video for this lecture has not been produced yet.
Reference Materials
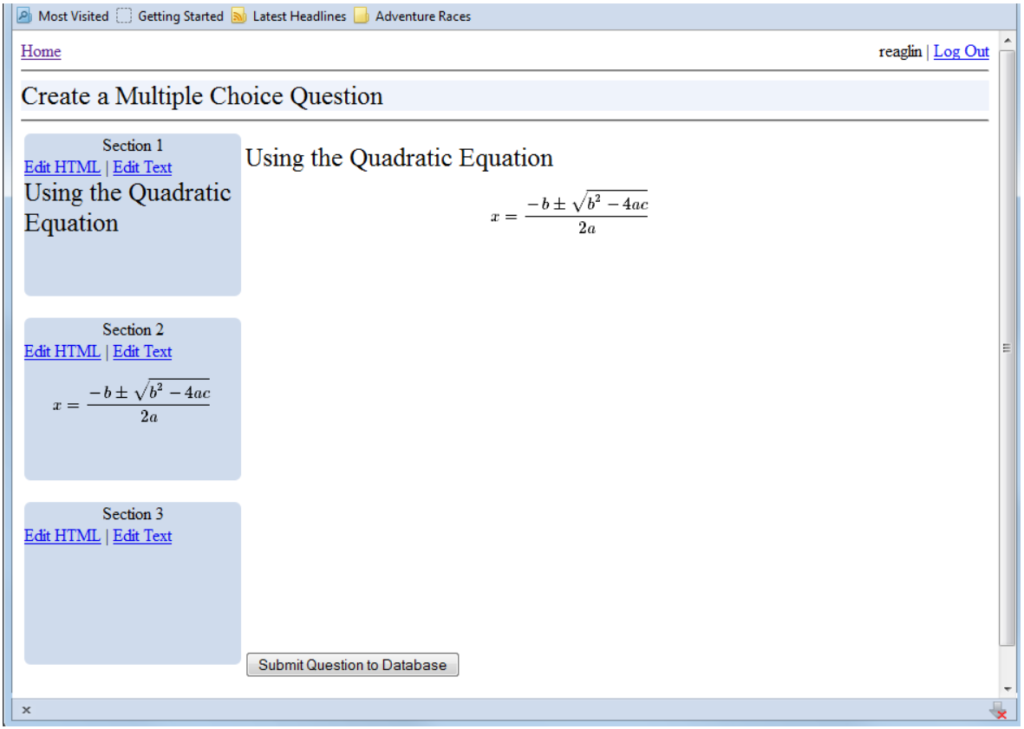
MCEquationEditor.aspx
<%@ Page Title="Create a Multiple Choice Question" Language="C#" MasterPageFile="~/LoggedIn.Master" AutoEventWireup="true" CodeBehind="CreateMCQuestion.aspx.cs" Inherits="MyDailyMath.Users.CreateMCQuestion" ValidateRequest="false" %>
<%@ Register Src="~/UserControls/ucEditPanel.ascx" TagPrefix="uc" TagName="EditPanel" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server">
<script type="text/javascript" src="http://cdn.mathjax.org/mathjax/latest/MathJax.js?config=TeX-AMS-MML_HTMLorMML">
</script>
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server">
<table>
<tr>
<td valign="top">
<uc:EditPanel runat="server" id="ucEP1" Header="Section 1"></uc:EditPanel><br />
<uc:EditPanel runat="server" id="ucEP2" Header="Section 2"></uc:EditPanel><br />
<uc:EditPanel runat="server" id="ucEP3" Header="Section 3"></uc:EditPanel><br />
</td>
<td>
<asp:TextBox ID="tbContentHTML" runat="server" TextMode="MultiLine" Width="600" Height="450" Visible="false"></asp:TextBox>
<ajaxToolkit:HtmlEditorExtender ID="html" runat="server" TargetControlID="tbContentHTML" DisplaySourceTab="true" >
</ajaxToolkit:HtmlEditorExtender>
<asp:TextBox ID="tbContentText" runat="server" TextMode="MultiLine" Width="600" Height="450" Visible="false" Enabled="true"></asp:TextBox>
<asp:Panel ID="PanelQuestion" runat="server" Width="600" Height="450" CssClass="QuestionDisplay" Visible="false" ScrollBars="Auto">
<asp:Literal ID="litQuestion" runat="server" ></asp:Literal>
</asp:Panel>
<br />
<asp:Button ID="btnSubmit" runat="server" Text="Enter Question Text" CssClass="QuestionButton"
onclick="btnSubmit_Click" Visible="false" />
</td>
</tr>
</table>
<br />
</asp:Content>
MCEquationEditor.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace MyDailyMath.Users
{
public partial class CreateMCQuestion : System.Web.UI.Page
{
public string mode { get { return Convert.ToString(Session["QEditMode"]);}
set {Session.Add("QEditMode", value); } } // html, text, done
public string editn { get { return Convert.ToString(Session["QEditN"]);}
set {Session.Add("QEditN", value); } } // 1, 2, or 3
protected void Page_Load(object sender, EventArgs e)
{
ucEP1.HtmlButtonClick += new UserControls.ucEditPanel.HtmlButtonClickHandler(ucEP1_HtmlButtonClick);
ucEP2.HtmlButtonClick += new UserControls.ucEditPanel.HtmlButtonClickHandler(ucEP2_HtmlButtonClick);
ucEP3.HtmlButtonClick += new UserControls.ucEditPanel.HtmlButtonClickHandler(ucEP3_HtmlButtonClick);
ucEP1.TextButtonClick += new UserControls.ucEditPanel.TextButtonClickHandler(ucEP1_TextButtonClick);
ucEP2.TextButtonClick += new UserControls.ucEditPanel.TextButtonClickHandler(ucEP2_TextButtonClick);
ucEP3.TextButtonClick += new UserControls.ucEditPanel.TextButtonClickHandler(ucEP3_TextButtonClick);
}
protected void btnSubmit_Click(object sender, EventArgs e)
{
btnSubmit.Visible = true;
btnSubmit.Text = "Submit Question to Database";
if (mode == "html")
{
if (editn == "1") ucEP1.Text = tbContentHTML.Text;
if (editn == "2") ucEP2.Text = tbContentHTML.Text;
if (editn == "3") ucEP3.Text = tbContentHTML.Text;
tbContentHTML.Visible = false;
tbContentText.Visible = false;
litQuestion.Text = fullContent();
PanelQuestion.Visible = true;
mode = "done";
return;
}
if (mode == "text")
{
if (editn == "1") ucEP1.Text = tbContentText.Text;
if (editn == "2") ucEP2.Text = tbContentText.Text;
if (editn == "3") ucEP3.Text = tbContentText.Text;
tbContentHTML.Visible = false;
tbContentText.Visible = false;
litQuestion.Text = fullContent();
PanelQuestion.Visible = true;
mode = "done";
return;
}
string final = fullContent();
// submit the question to database here
}
private string fullContent()
{
return ucEP1.Text + ucEP2.Text + ucEP3.Text;
}
private void setHTMLEdit(string n)
{
tbContentHTML.Visible = true;
PanelQuestion.Visible = false;
tbContentText.Visible = false;
btnSubmit.Visible = true;
btnSubmit.Text = "Submit HTML for Section " + n;
mode = "html";
editn = n;
}
private void setTextEdit(string n)
{
tbContentHTML.Visible = false;
PanelQuestion.Visible = false;
tbContentText.Visible = true;
btnSubmit.Visible = true;
btnSubmit.Text = "Submit Text for Section " + n;
mode = "text";
editn = n;
}
protected void ucEP1_HtmlButtonClick(object sender, EventArgs e)
{
setHTMLEdit("1");
tbContentHTML.Text = ucEP1.Text;
}
protected void ucEP2_HtmlButtonClick(object sender, EventArgs e)
{
setHTMLEdit("2");
tbContentHTML.Text = ucEP2.Text;
}
protected void ucEP3_HtmlButtonClick(object sender, EventArgs e)
{
setHTMLEdit("3");
tbContentHTML.Text = ucEP3.Text;
}
protected void ucEP1_TextButtonClick(object sender, EventArgs e)
{
setTextEdit("1");
tbContentText.Text = ucEP1.Text;
}
protected void ucEP2_TextButtonClick(object sender, EventArgs e)
{
setTextEdit("2");
tbContentText.Text = ucEP2.Text;
}
protected void ucEP3_TextButtonClick(object sender, EventArgs e)
{
setTextEdit("3");
tbContentText.Text = ucEP3.Text;
}
}
}
Additional Information
http://www.mathjax.org/ – MathJax JavaScript library
http://www.asp.net/ajax – AJAX Control Toolkit
http://visualstudiogallery.msdn.microsoft.com/27077b70-9dad-4c64-adcf-c7cf6bc9970c/ – NuGet Package Manager
Part of theĀ Case Study – My Daily Math