Even though we still have many features of Python, it is now time to start looking at the many libraries that are available to Python. First there are all the standard libraries that you can review at https://docs.python.org/3/library/ . Let’s first look at the built-in stuff you should know at this point;
- Data types; https://docs.python.org/3/library/stdtypes.html#numeric-types-int-float-complex
- Data type integer (int)
- Data type string (str)
- Data type float
- Data Type list (also called array here)
- Boolean operators: https://docs.python.org/3/library/stdtypes.html#boolean-operations-and-or-not
- Built in functions: https://docs.python.org/3/library/functions.html
- input()
- len()
- print()
- range()
- str()
- Programming concepts
- variables: assigning values to variables and lists
- conditional: if then elif
- looping: for loop
- looping: while loop
- function (writing and calling functions)
With this as a background you are ready to jump into much more complex programming. This means moving on to using some of the more complex libraries. Some of these come with the Anaconda distribution.
The math library – https://docs.python.org/3/library/math.html
As an engineer this will probably be your most useful library – it contains various math functions and everyone should get familiar with it, if for no reason other than it is very important to know what math function are there. An example of using the math library is below.
import math
math.gcd(24,16)
Out[5]: 8
You may wish to use a library that is not included in the Anaconda download, in which case you will need to use the conda installation manager. This is a command line tool, so get used to issuing command line commands (a lost art). On Windows you can access the conda prompt through the Anaconda Menu, Anaconda prompt. Open it and type conda.
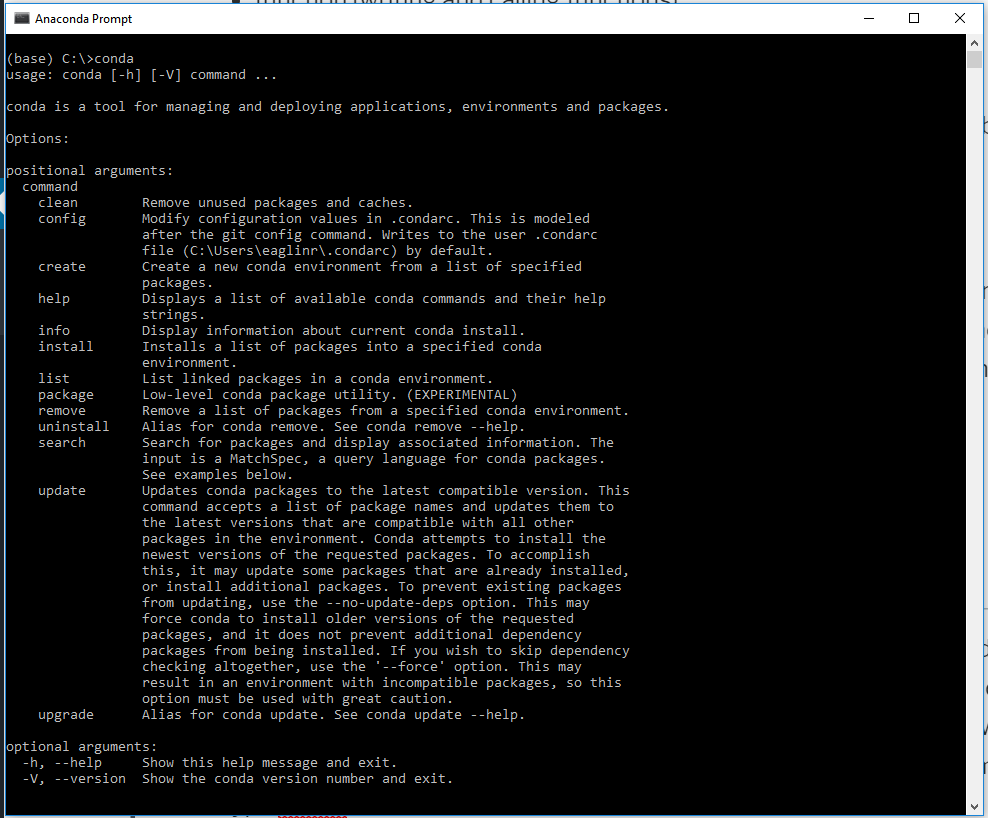
The command line for installing a package is simple, simply replace package_name with the name of the package you wish to install. Conda will find dependencies and install all the packages necessary for the package you wish to install. Typically if you have found the package the command line requirements will be in the documentation of the package.
c:\>conda install package_name
For more information on using conda see the documentation at https://docs.conda.io/projects/conda/en/latest/user-guide/getting-started.html