Previous – Setting up the IDE
Getting Started
Let’s get started with variables, just like in algebra variables carry values and can be assigned to these values. There are 3 primary types of variables you will use in programming in Python; integers, floating point numbers, and strings (text). Here we will define these and look at them in the variable explorer. There are naming rules for variables – but for now we will simply use simple strings and groups of letters.

To get an idea of how these work, let’s try adding different combinations of these types of variables.
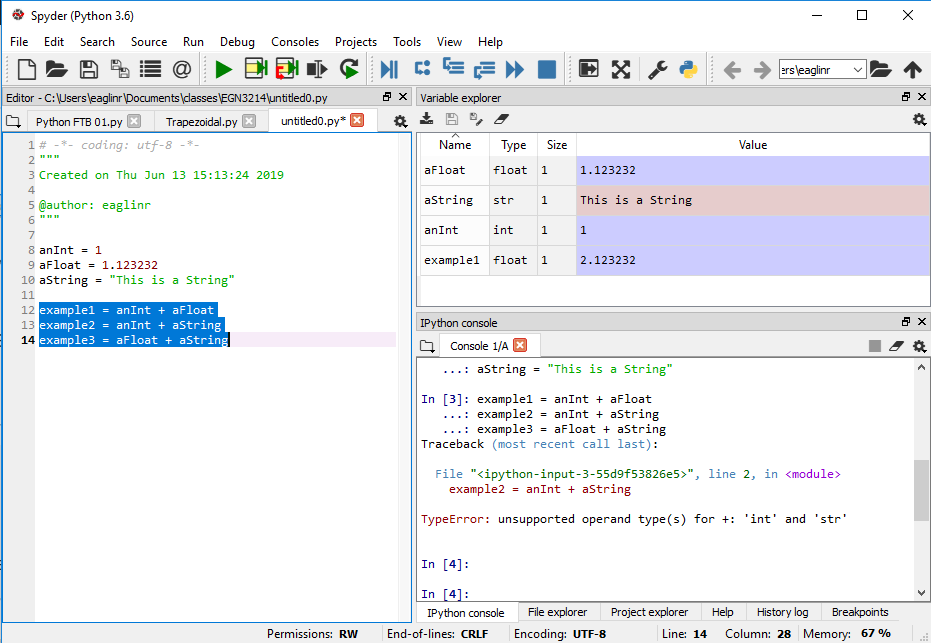
As you might expect integers and floats add just fine. We do run into a problem though when we try to add the integer to the string, the operand + is not supported for types ‘int’ and ‘string’ – basically saying we cannot add them together. Also it is worth noting that when we added the float and the integer, the result was a float (hopefully nobody is surprised by either of these).
There are cases where you may want to add a string and an integer (basically add the value of the int to the string as text). This can be done and pretty easily – it simply requires casting one variable to another type of variable. Here is where we “learn to learn”
Asking Google for Help
Armed with the knowledge that we are using Python and we are doing an operation called casting, we can ask Google to help us find the correct syntax to do this. In programming language matters – the operations have specific names and knowing these names can come in real handy when trying to write programs. So let’s ask Google….
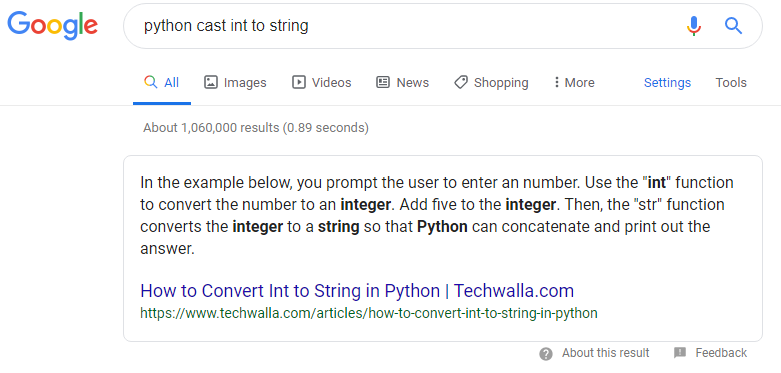
This may look like a really simple request and solution, but there is much more to it. First the language python cast in to string is very specific and allows us to get very specific results, which if we do not want to spend a lot of time searching through the search results is important.
Also important is that we understand the answer. In this case there are now 2 new terms that are important; concatenate and the str function. This is important in that we now know that by converting an integer to a string we can concatenate it to another string. In other words the string is simply “tacked on” to the existing string and that is how strings are added.
Secondly it introduces the concept of the str function, something we will need to know how to apply. So let’s try this in code.
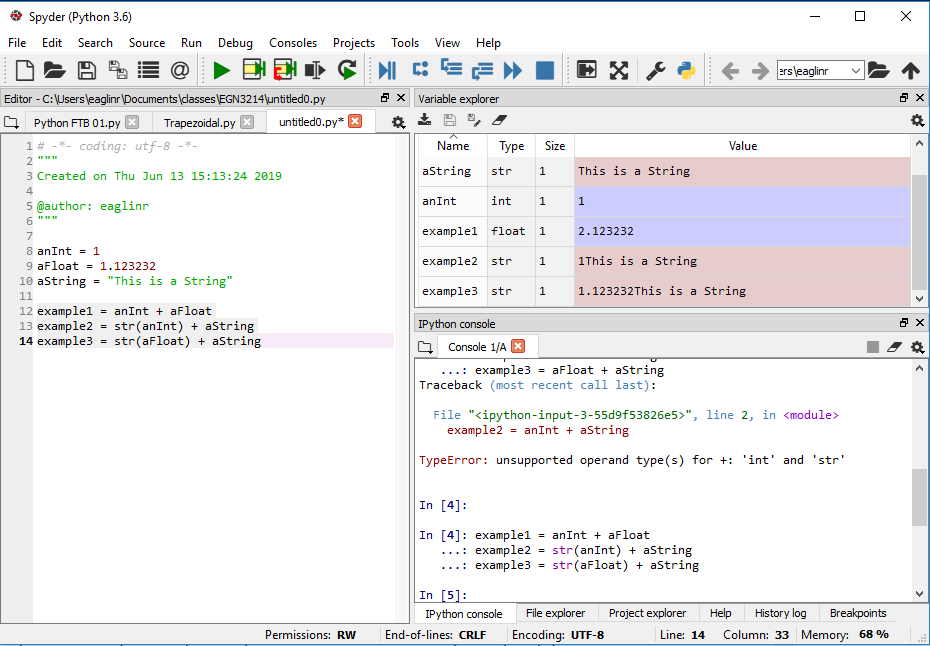
Now notice what happened. The integer anInt was converted to a string and concatenated onto aString and assigned to example2. Same for the float and example3. You can see the results in the Variable Explorer. This also introduces the concept of the function.
Functions (conceptually)
A function is also code that takes inputs, performs a task, and optionally returns something. Python has a lot of built-in functions. Some will be very useful, like the print function and input function.
Functions have these key elements;
- A name – in the example the name is str.
- Input parameters or Arguments – in the example the input parameter is the integer that was inside the brackets. In nearly all languages the input parameters of a function are inside parentheses ( ).
- Output or Return Value – This is optional as functions do not have to return anything. Function in Python can return a single value or multiple values (we will discuss much later)
Functions can also go by different names and these have specific meanings in different situations (method, procedure, routine, operation, and command). All of these are still functions in that they have a name, do something and can accept arguments and return something.
Built-in Python Functions
The list of all Python built-in functions is available in the Python documentation at https://docs.python.org/3/library/functions.html . This list has some simple function that you will use all the time like str(), print(), range(), and len(). You should look at this documentation and bookmark it for reference. Until you become proficient it is common the find yourself trying to remember that specific function you need.
You should look through this documentation. You will notice that some of the function like str() have the word class before the name in documentation. We are not going to worry about what that means right now, later we will look at what this means (when we look at classes and instantiation). For now it should suffice that you have some useful functions built in to Python that do useful things.
Arguments – it is worth noting at this time that some of the functions can take different number and types of arguments. This is a really handy thing in programming called polymorphism. You don’t need to remember that word for now – but polymorphism will make your programming life easier. It allows us to use the same function name to do essentially the same thing with different arguments. You’ve already used it. In our example the str() function took an integer and returned a string. It also took a float and returned a string. We never even thought about the fact that the arguments were different, but the programmers of the function did and decided that the str() should convert whatever you give it into a string if at all possible or logical to do so.
example1 = anInt + aFloat
example2 = str(anInt) + aString
example3 = str(aFloat) + aString
Creating your own function
You can create your own functions – and this is an amazingly handy thing to be able to do. Let’s do this by example;
def addAsString(var1, var2):
str1 = str(var1)
str2 = str(var2)
str3 = str1 + str2
return str3
example4 = addAsString(anInt, aString)
example5 = addAsString(anInt, aFloat)
In this example we define the function addAsString that accepts 2 arguments. It will convert both arguments to strings and return the concatenation.
We call the function and the return values are assigned to example4 and example5. We can print out the values of the returns from the console window by simply typing in the print function with the return variables as arguments as shown.
def addAsString(var1, var2):
str1 = str(var1)
str2 = str(var2)
str3 = str1 + str2
return str3
example4 = addAsString(anInt, aString)
example5 = addAsString(anInt, aFloat)
print(example4)
1This is a String
print(example5)
11.123232
It is worth noting that the value assigned to example5 (variable) is the string concatenation of the integer (1) and the float (1.123232).
Additional Resources
Programiz on Variables – https://www.programiz.com/python-programming/variables-constants-literals
Programiz on Functions – https://www.programiz.com/python-programming/function
Next: Conditional Logic